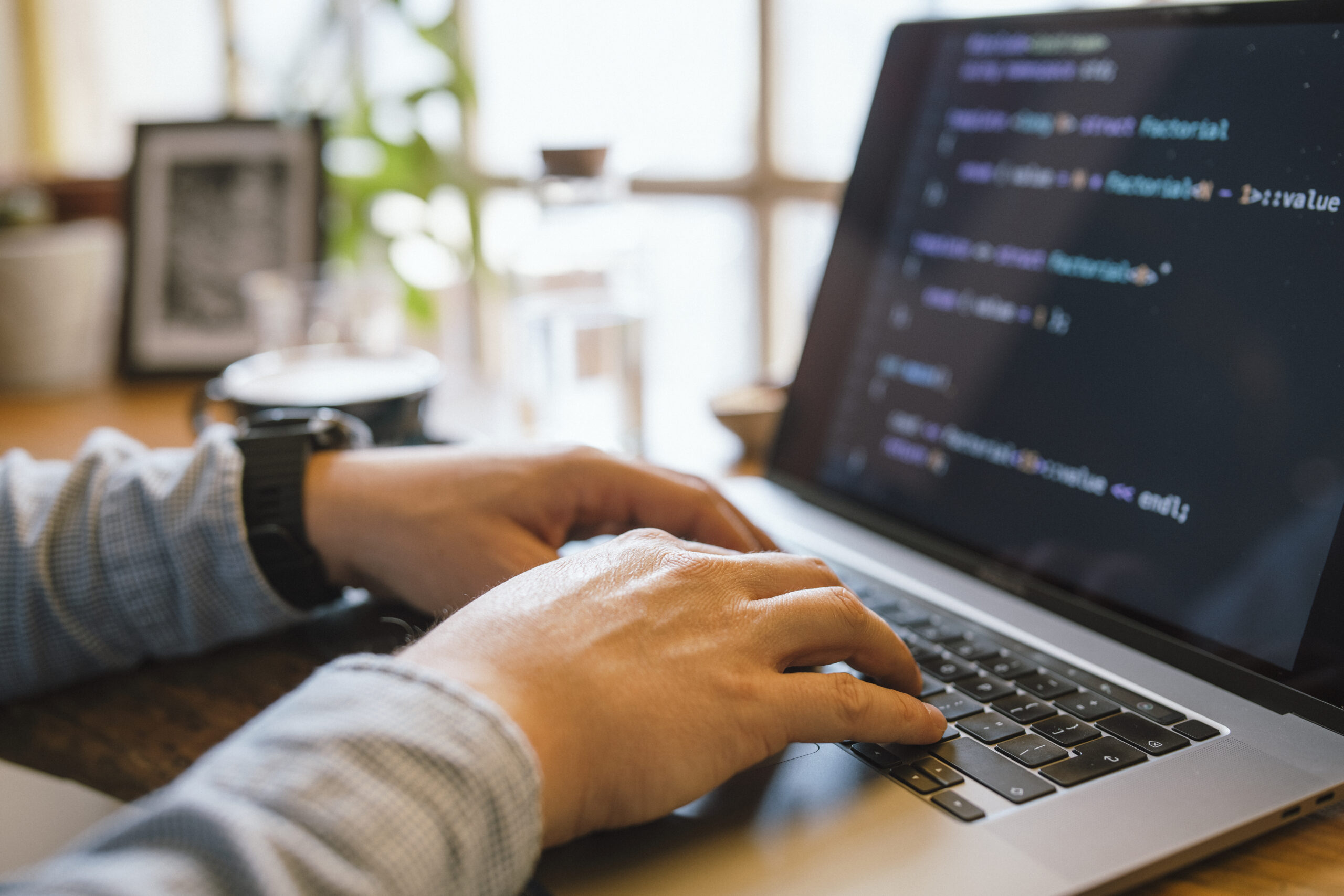
Debugging is The most critical — but typically forgotten — skills inside a developer’s toolkit. It is not nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to think methodically to solve problems efficiently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of frustration and dramatically improve your productivity. Here are quite a few procedures that can help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest techniques developers can elevate their debugging skills is by mastering the applications they use on a daily basis. Even though creating code is just one Element of progress, figuring out the way to interact with it successfully throughout execution is Similarly vital. Present day advancement environments come Geared up with highly effective debugging capabilities — but several developers only scratch the surface of what these instruments can do.
Get, for example, an Built-in Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments help you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in many cases modify code about the fly. When utilised properly, they Enable you to notice precisely how your code behaves during execution, which is a must have for tracking down elusive bugs.
Browser developer applications, such as Chrome DevTools, are indispensable for entrance-conclude developers. They enable you to inspect the DOM, monitor network requests, perspective actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can transform aggravating UI challenges into manageable duties.
For backend or procedure-level developers, instruments like GDB (GNU Debugger), Valgrind, or LLDB offer deep Handle in excess of running processes and memory management. Mastering these tools might have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Handle programs like Git to be familiar with code history, uncover the precise instant bugs were being introduced, and isolate problematic modifications.
In the end, mastering your applications means going beyond default settings and shortcuts — it’s about establishing an personal expertise in your advancement natural environment so that when problems arise, you’re not dropped in the dead of night. The higher you already know your applications, the greater time you may shell out fixing the actual dilemma rather than fumbling via the process.
Reproduce the Problem
Probably the most crucial — and often overlooked — ways in helpful debugging is reproducing the condition. In advance of leaping in to the code or creating guesses, developers have to have to produce a reliable setting or situation exactly where the bug reliably appears. Without reproducibility, correcting a bug will become a match of possibility, usually leading to squandered time and fragile code improvements.
The first step in reproducing a dilemma is accumulating as much context as possible. Check with inquiries like: What actions brought about the issue? Which ecosystem was it in — growth, staging, or creation? Are there any logs, screenshots, or mistake messages? The greater depth you've got, the less difficult it gets to be to isolate the exact conditions underneath which the bug happens.
Once you’ve gathered adequate information, seek to recreate the situation in your neighborhood atmosphere. This may necessarily mean inputting a similar info, simulating identical consumer interactions, or mimicking procedure states. If The problem seems intermittently, take into account writing automatic tests that replicate the edge conditions or state transitions included. These checks not just assistance expose the issue and also stop regressions Sooner or later.
In some cases, The problem can be atmosphere-certain — it'd happen only on specific running units, browsers, or underneath particular configurations. Making use of applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the trouble isn’t merely a move — it’s a mindset. It needs endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are presently halfway to repairing it. Using a reproducible situation, You need to use your debugging instruments additional proficiently, take a look at opportunity fixes properly, and connect additional clearly with your team or users. It turns an abstract criticism right into a concrete problem — and that’s wherever builders thrive.
Study and Comprehend the Error Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really study to deal with error messages as immediate communications with the technique. They usually tell you what precisely took place, in which it occurred, and at times even why it happened — if you know the way to interpret them.
Start off by studying the information thoroughly and in whole. A lot of developers, specially when below time pressure, look at the very first line and straight away start off creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root bring about. Don’t just copy and paste mistake messages into engines like google — study and fully grasp them very first.
Crack the error down into sections. Can it be a syntax error, a runtime exception, or maybe a logic error? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some mistakes are obscure or generic, As well as in those circumstances, it’s important to look at the context by which the error occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These frequently precede more substantial challenges and supply hints about possible bugs.
In the end, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Just about the most effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s happening under the hood without having to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic data for the duration of growth, Information for common events (like successful get started-ups), Alert for prospective problems that don’t crack the appliance, ERROR for actual complications, and Deadly once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant knowledge. A lot of logging can obscure important messages and decelerate your program. Concentrate on vital functions, state variations, enter/output values, and significant choice details within your code.
Structure your log messages Plainly and regularly. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to track how variables evolve, what problems are met, and what branches of logic are executed—all with no halting This system. They’re Specially valuable in creation environments where by stepping by means of code isn’t probable.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. Using a very well-thought-out logging technique, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and dependability within your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a kind of investigation. To proficiently detect and repair bugs, developers need to tactic the procedure like a detective solving a mystery. This attitude will help stop working complex problems into manageable elements and comply with clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the symptoms of the problem: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, gather as much related info as you'll be able to without having jumping to conclusions. Use logs, check instances, and user experiences to piece alongside one another a transparent photo of what’s occurring.
Up coming, sort hypotheses. Check with by yourself: What may be triggering this conduct? Have any modifications recently been made into the codebase? Has this issue occurred right before less than related conditions? The objective is to slender down options and recognize possible culprits.
Then, test your theories systematically. Seek to recreate the challenge within a managed natural environment. Should you suspect a specific functionality or element, isolate it and verify if The problem persists. Like a detective conducting interviews, talk to your code inquiries and let the effects direct you nearer to the truth.
Pay near interest to compact information. Bugs often cover within the the very least anticipated sites—just like a lacking semicolon, an off-by-one particular mistake, or a race affliction. Be comprehensive and patient, resisting the urge to patch The difficulty with no absolutely comprehension it. Temporary fixes may possibly disguise the true challenge, only for it to resurface later on.
Lastly, continue to keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can preserve time for future concerns and assistance Other individuals have an understanding of your reasoning.
By considering just like a detective, builders can sharpen their analytical competencies, method troubles methodically, and come to be more effective at uncovering hidden troubles in elaborate methods.
Publish Assessments
Crafting tests is one of the best strategies to transform your debugging skills and Over-all enhancement performance. Tests not just aid catch bugs early but in addition function a security Internet that provides you assurance when earning changes on your codebase. A perfectly-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly where and when an issue occurs.
Start with unit tests, which concentrate on person functions or modules. These little, isolated tests can quickly reveal whether a selected bit of logic is Performing as predicted. Each time a examination fails, you right away know in which to search, considerably decreasing the time used debugging. Device exams are Primarily handy for catching regression bugs—troubles that reappear right after previously remaining fastened.
Following, integrate integration checks and conclusion-to-stop tests into your workflow. These assistance be sure that different elements of your software operate collectively smoothly. They’re particularly handy for catching bugs that take place in sophisticated systems with various elements or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and less than what problems.
Creating assessments also forces you to Imagine critically about your code. To check a attribute correctly, you would like to grasp its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally sales opportunities to better code framework and read more fewer bugs.
When debugging a difficulty, composing a failing exam that reproduces the bug may be a strong starting point. After the take a look at fails regularly, it is possible to focus on repairing the bug and enjoy your test move when The problem is fixed. This method makes sure that the exact same bug doesn’t return in the future.
In a nutshell, producing checks turns debugging from a aggravating guessing match right into a structured and predictable process—assisting you catch additional bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your intellect, cut down frustration, and often see The difficulty from the new standpoint.
When you're too close to the code for too long, cognitive exhaustion sets in. You might start overlooking noticeable faults or misreading code that you choose to wrote just several hours before. With this condition, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report discovering the foundation of a challenge once they've taken time for you to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, Specially in the course of lengthier debugging classes. Sitting before a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away helps you to return with renewed Strength along with a clearer mentality. You could possibly abruptly see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
When you’re stuck, a fantastic rule of thumb should be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that point to maneuver all around, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily beneath limited deadlines, however it essentially results in speedier and more effective debugging Eventually.
To put it briefly, using breaks will not be a sign of weak point—it’s a sensible strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Every bug you experience is much more than simply A short lived setback—It is a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, every one can teach you one thing worthwhile when you take the time to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of important queries after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught before with superior techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots within your workflow or being familiar with and help you build much better coding patterns going ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent faults—which you could proactively stay away from.
In team environments, sharing what you've figured out from a bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, serving to Other folks avoid the exact situation boosts team performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In lieu of dreading bugs, you’ll commence appreciating them as crucial parts of your growth journey. In the end, a lot of the greatest builders usually are not those who create great code, but people who repeatedly discover from their faults.
In the end, Every single bug you fix adds a completely new layer for your talent established. So up coming time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more capable developer as a consequence of it.
Conclusion
Bettering your debugging techniques requires time, follow, and tolerance — but the payoff is huge. It can make you a far more efficient, assured, and able developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.